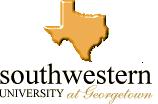 |
Conditionals Part II
|
Ifs within ifs
-
A nested if is an if embedded in another if
-
consider that if is a statement, and so it can go in the statement
part of:
if (condition)
statement
if (num > 0) { // start > 0
if (num < 10)
System.out.print ("Small");
else
System.out.print ("Large");
System.out.print (" and positive");
} // end > 0
else
System.out.println ("Zero or negative");
Note, that braces are necessary
if (temperature > 90)
if (humidity > 80)
System.out.println ("Hot and humid");
else
System.out.println ("Comfortable");
This is called a dangling else; else always matches the most recent unmatched
if, regardless of indentation.
Another primitive data type: boolean
-
Expressions like 5+3 return an int value
-
Expressions lik 5 < 3 return boolean values
-
boolean models a truth table
-
it has only two possible values: true or false
-
Like all primitive data types, can
-
declare boolean variable
-
assign boolean values to boolean variables
-
construct boolean expressions
-
pass booleans as arguments
-
weatherChannel.reportWeather (isHot);
-
return boolean
-
if (weatherChannel.haveHurricaneWarning ("SC")) ...
-
but, anything resulting in a boolean can be used in a condition
if (isHot) {
System.out.println ("Iced tea, please.");
}
Predicate methods
-
many classes have a method that returns a boolean, and can be used as a
condition
-
For example, the class String has an equals method
if (s1.equals (s2))
System.out.println ("s1 has the same characters as s2");
note, to compare two strings ALWAYS use equals method
-
never use s1 == s2
-
what does it mean?
consider following class:
class Name {
private String first;
private String last;
...
public boolean equals (Name n) {
if (first.equals (n.first)) {
if (last.equals (n.last)) {
return true;
} else
return false;
} else
return false;
}
...
}
Digression:
-
Inside a class, any reference to another class can use private information
-
Information is invisible to objects of different classes, not the same
class
-
i.e., even thought first and last are private, they can be seen by other
Name objects (but not, for example, a Dog object).
Logical operators
-
Notice in the example equals above, that one Name object is equal to another
if both the first and last names are equal.
-
Java provides a logical operator, &&, that represents and.
if (first.equals (n.first) && last.equals (n.last)) ...
This will execute the true part iff the first names and last names contain
the same characters
A && B is true only if both operands are true
There is also a logical or, ||, that returns true if one or the other operand
is true
first.equals (n.first) || last.equals (n.last)
This would be true for Brad Schmerl and Brad Pitt, or Bradley Schmerl and
Robert Schmerl.
Finally, there is a logical not operator, !, that has a single operand.
Summary
A |
B |
A && B |
A || B |
! A |
true |
true |
true |
true |
false |
true |
false |
false |
true |
false |
false |
true |
false |
true |
true |
false |
false |
false |
false |
true |
Larger logical expressions
class Time {
private int hours;
private int minutes;
public boolean isBefore (Time t) ...
...
}
Using the above example, how can we tell if one time is before another?
e.g., is 12:30 before 11:30? 15:20 before 15:25?
There are two cases: if the hours are less, then one is before the other,
if they're the same, then one time is before the other if there are less
minutes. Thus, we can write the expression:
if (this.hours < t.hours) {
return true;
} else if (this.hours == t.hours && this.minutes < t.minutes) {
return true;
} else {
return false;
}
These if statements can be merged. We are returning the same thing if either
the first or second condition is true.
if (this.hours < t.hours || (this.hours == t.hours && this.minutes < t.minutes)) {
return true;
}
Based on material by: Alan
Kaplan of Clemson Univesity
Revisions responsibility of Barbara
Boucher Owens
Last modified:25 Feb 2000